2020. 4. 21. 01:32ㆍCSS
## SCSS:
### Preprocessors and Set Up
1. SCSS는 기본적으로 css의 전처리기
- css의 전처리기 (preprocessor): SCSS -> 컴파일 -> CSS 코드
- css의 다른 preprocessor: stylus, less 등
2. SCSS가 Sass의 공식 syntax (둘이 같다고 생각하면 됨)
3. 컴파일하거나 빌드하는 단계가 필요
- gulp나 webpack을 이용
- 만들어진 gulp / webpack은 예시) styles.scss 등의 특정 파일을 css파일로 컴파일
- index.html은 컴파일된 css파일을 가리키고 있어야 함 (브라우저는 scss를 이해 못하기 때문)
### Concepts
1. Variables
- scss파일들이 들어 있는 폴더에 variables를 담을 scss 파일 생성
- 파일명 앞에 _ (underbar) 붙여서 만듦: css로 컴파일 하고싶지 않은 파일이라는 의미
- 변수 만들기
$[변수명]: 값
/* ex) $background: 'red' */
2. Nesting
- targeting 하는 요소를 더욱 명확하게 보여줌 (가독성 up)
- 또, 부모-자식 요소간 스타일 적용할 때 중복을 피하게 해 줌
.box {
margin-top: 20px;
&:hover{
background-color: green;
}
h2 {
color: blue;
}
button {
color: red;
}
}
- 위와 같은 형식으로 적어 .box / .box h2 / .box button 따로따로 스타일 설정하는 것을 피할 수 있음
- 가상선택자는 중복되는 부분 & 으로 표현
- nest 안에 다시 nest 해서 표현가능
3. Mixins
- scss functionality를 재사용할 수 있도록 해줌
/* _mixins.scss */
@mixin title {
color: blue;
font-size: 30px;
margin-bottom: 20px;
}
/* 아래의 형식으로 사용
@mixin [mixin 이름] {
속성명: 속성값;
}
*/
/* styles.scss */
@import "_mixins";
h1 {
@include title();
}
/* 아래의 형식으로 사용
[적용할 요소] {
@include [mixin 이름]
}
*/
- title이라는 mixin에서 style들을 지정해 주고, h1에서 적용시켜 주면 h1의 style이 mixin에서 지정한 내용이 됨
- 이런 식으로 함수처럼 표현할 수도 있음
: 큰 틀은 같지만 디테일한 부분을 다르게 하고 싶을 때
/* _mixins.scss */
@mixin link($color) {
text-decoration: none;
display: block;
color: $color;
}
/* styles.scss */
@import "_mixins";
a {
margin-bottom: 10px;
&:nth-child(odd) {
@include link(blue);
}
&:nth-child(even) {
@include link(red);
}
}
- styles.scss에서 매개변수로 색상명을 넘기고 있고,
- link라는 mixin에서 받은 색상명을 가지고 글자색을 지정해주고 있음
- 또, mixin 안에서 if문을 사용하거나 변수 대신 텍스트를 mixin으로 넘길 수도 있음
/* _mixins.scss 파일 */
@mixin link($word) {
text-decoration: none;
display: block;
@if $word == "odd" { /* 서로 같은지 비교할 때 == 사용하는 것 주의 (=== 사용시 오류) */
color: blue;
} @else {
color: red;
}
}
/* styles.scss 파일 */
@import "_mixins";
a {
margin-bottom: 10px;
&:nth-child(odd) {
@include link("odd");
}
&:nth-child(even) {
@include link("even");
}
}
- styles.scss에서 매개변수로 텍스트를 넘기고 있고,
- mixin 안에서 if문을 이용해 매개변수로 받은 텍스트에 따라 글자색이 달라지도록 하고 있음
4. Extend
- 다른 코드를 확장하거나 코드 재사용이 용이하게 함
- 공통적인 것들을 extend 안에 넣어 재사용함
/* 아래의 형식으로 사용 */
%[extend 이름] {
속성명: 속성값;
}
/* _buttons.scss (extend 요소 들어 있는 파일) */
%button {
font-family: inherit;
border-radius: 7px;
font-size: 12px;
text-transform: uppercase;
padding: 5px 10px;
background-color: yellowgreen;
font-weight: 500;
}
/* styles.scss 파일 */
@import "_buttons";
a {
@extend %button;
text-decoration: none;
}
button {
@extend %button;
border: none;
}
- _buttons.scss 안에 공통적인 스타일들을 넣고
- styles.scss에서 extend로 %button 사용, 개별 설정들을 적용해 주었음
5. Responsive Mixins
- content: mixin에 스타일을 전달
/* _mixins.scss 파일: */
@mixin anchor {
color: red;
@content;
}
/* styles.scss 파일 */
@import "_mixins";
a {
@include anchor {
text-decoration: none;
}
}
- text-decoration: none 부분이 content가 되어 mixin의 content가 됨
- content는 media query와 함께 사용될 수 있음
/* _mixins.scss 파일 */
$minIphone: 500px;
$maxIphone: 690px;
@mixin responsive($device) {
@if $device == "iphone" {
@media screen and (min-width: $minIphone) and (max-width: $maxIphone) {
@content;
}
} @else if $device == "iphone-l" {
@media screen and (min-width: $minIphone) and (max-width: $maxIphone) (orientation: landscape) {
@content;
}
}
}
/* styles.scss 파일 */
@import "_mixins";
h1 {
color: red;
@include responsive("iphone") {
color: yellow;
}
@include responsive("iphone-l") {
font-size: 60px;
color: yellow;
}
}
- response mixin에서는 device를 매개변수로 받아, 각 device에 맞는 미디어스크린 효과를 지정해주고 있음
- 또, 미디어스크린 안의 내용은 content로 styles에서 받아오고 있음
- 이를 적용했을 때:
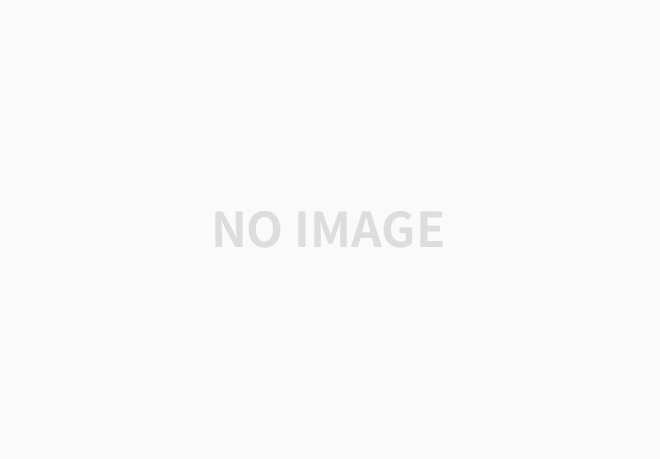
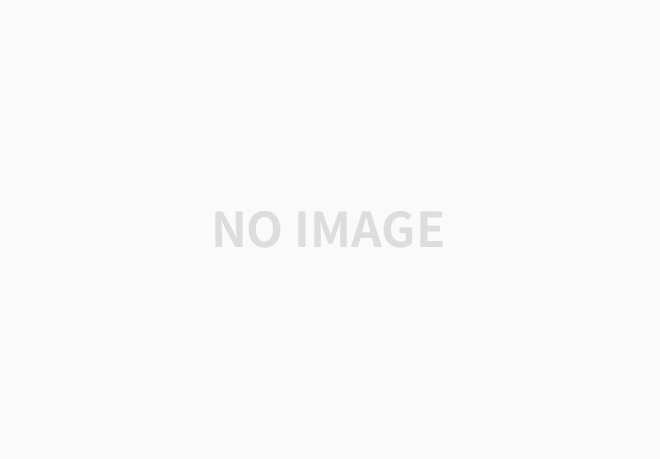
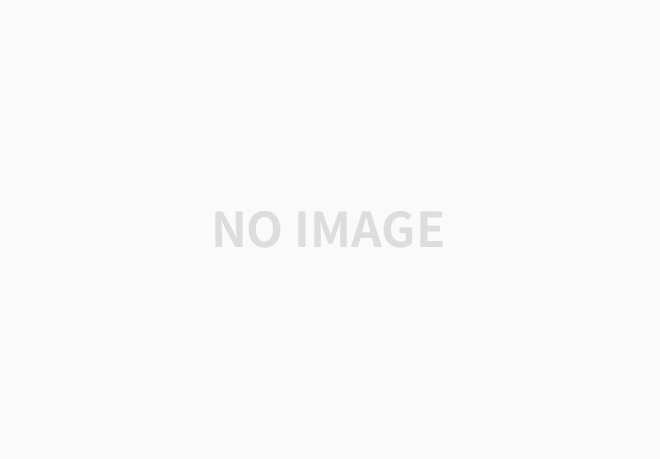
'CSS' 카테고리의 다른 글
Grid Concepts (0) | 2020.04.30 |
---|