2020. 4. 30. 21:21ㆍCSS
## Grid:
### Why we use CSS-Grid?
#### Flexbox로는 Grid를 만들기가 어렵다
flex box에서는:
1. 요소들을 정렬한 뒤, 첫째줄을 제외한 다른 요소들의 공백을 맞춰주려면 요소마다 개별적으로 margin 등을 적용해야 한다.
2. 요소들을 순서대로 격자모양으로 배치하는 것이 어렵다.
- flex box에서 justify-content / align-items: space-between 을 적용했을 때:
<HTML>
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="style.css" />
<title>Document</title>
</head>
<body>
<div>1</div>
<div>2</div>
<div>3</div>
<div>4</div>
<div>5</div>
</body>
</html>
<CSS>
body {
display: flex;
flex-direction: row;
flex-wrap: wrap;
justify-content: space-between;
}
div {
width: 200px;
height: 200px;
background-color: dodgerblue;
margin: 10px;
}
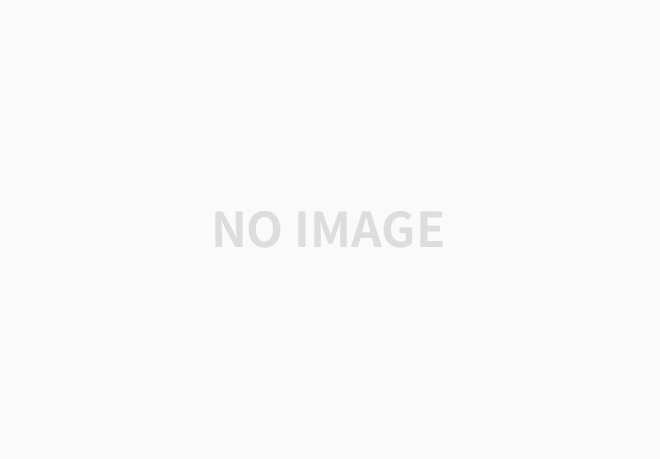
- 윗줄에는 차례로 요소가 들어가지만 아랫줄에는 양옆으로 요소들이 떠서 보이는 것과 같은 문제가 존재
### Grid Conceps
1. 기본적으로 부모 요소에서 일어난다
2. Grid에는 row와 column이 존재
3. row와 row 사이, column과 colunm 사이의 공간을 line이라고 부름
### properties
1. grid-template-columns: 각 column들의 너비를 지정
body {
display: gird;
grid-template-columns: 200px, 200px, 200px;
}
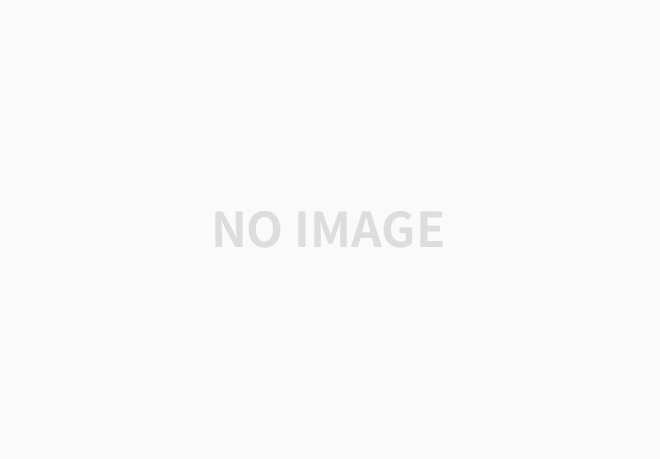
- 적어준 갯수의 너비만큼의 column 갯수가 생김
- 아랫줄로 내려간 column들은 윗줄의 같은 위치 요소와 같은 너비를 가짐
body {
display: gird;
grid-template-columns: repeat(3, 200px);
}
- repeat에 지정할 갯수, 너비 넣어 반복 없앨 수 있음
- auto 지정시 화면의 전체 크기 사용
body {
display: gird;
grid-template-columns: linename1 1fr linename2 1fr linename3;
}
- line들의 이름 지정 가능 (line은 각 column 사이사이를 말함)
- pixel 값 지정 대신 fr(fraction) 사용 (grid 안에서 가질 수 있는 공간 크기만큼을 차지)
- 모든 요소를 1fr로 지정했을 경우 너비가 모두 같아짐
- 4fr 1fr 1fr의 경우에는 첫번째 요소가 나머지의 4배만큼 커짐
body {
display: grid;
grid-template-columns: 1fr 4fr 1fr;
padding: 10px;
}
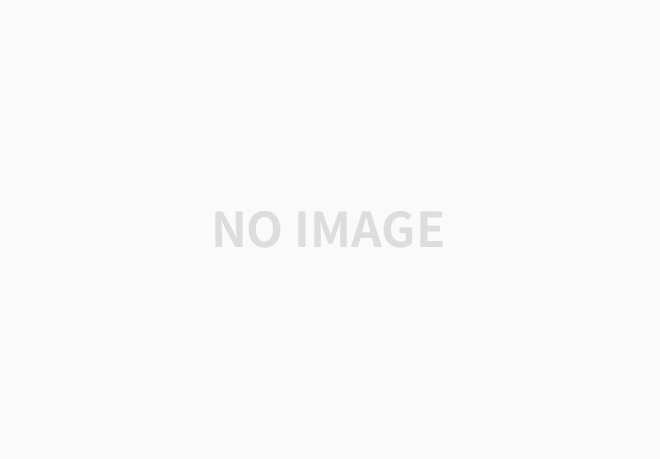
2. grid-template-rows: 각 row들의 높이를 지정
body {
display: gird;
grid-template-rows: 200px, 200px;
}
- 적어준 갯수의 높이만큼의 row 갯수가 생김
- 같은 row의 요소들끼리는 같은 높이를 가짐
body {
display: gird;
grid-template-rows: repeat(2, 200px);
}
- repeat에 지정할 갯수, 높이 넣어 반복 없앨 수 있음
- auto 지정시 화면의 전체 크기 사용
- line들의 이름 지정 가능
- pixel 값 지정 대신 fr(fraction) 사용 (grid 안에서 가질 수 있는 공간 크기만큼을 차지)
- rows에 fr 적용할 경우에는 grid의 높이가 꼭 필요(지정해주지 않으면 0)
3. column-gap: 각 column 사이의 간격을 지정
4. row-gap: 각 row 사이의 간격을 지정
5. gap: column-gap + row-gap
body {
display: grid;
width: 90vw;
height: 50vh;
grid-template-columns: repeat(3, 1fr);
grid-template-rows: repeat(2, 1fr);
gap: 10px;
}
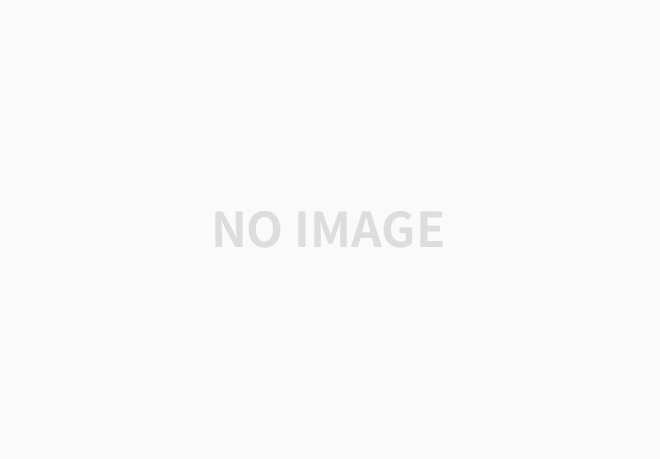
6.grid-template-areas: 직접 template 지정 가능
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="style.css" />
<title>Document</title>
</head>
<body>
<div class="header"></div>
<div class="content"></div>
<div class="nav"></div>
<div class="footer"></div>
</body>
</html>
body {
display: grid;
grid-template-columns: repeat(4, 100px);
grid-template-rows: repeat(4, 100px);
grid-template-areas:
"header header header header"
"content content content nav"
"content content content nav"
"footer footer footer footer";
}
- 빈 공간을 만들려면 template의 이름 대신 '.' 로 지정
7. grid-area: grid-template-areas에 적용할 이름 설정
- 자식 요소에 지정
.header {
background-color: #2ecc71;
grid-area: header;
}
.content {
background-color: #3498db;
grid-area: content;
}
.nav {
background-color: #8e44ad;
grid-area: nav;
}
.footer {
background-color: #f39c12;
grid-area: footer;
}
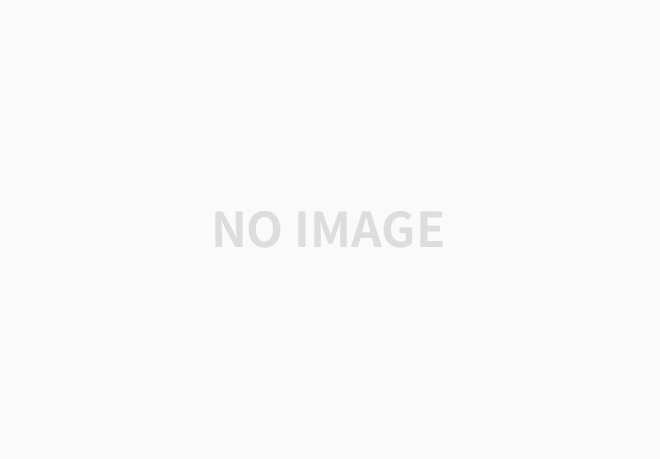
8. grid-column-start: column이 어디에서부터 시작할지 지정
- 자식 요소에 지정
- column 얘기 X, Line 얘기
9. grid-column-end: column이 어디에서 끝날지 지정
- 자식 요소에 지정
- column 얘기 X, Line 얘기
grid-column-start: 1;
grid-column-end: 2;
- 위의 예시의 경우 해당 요소는 첫번째 줄에서 시작해서 두번째 줄에서 끝난다는 뜻
10. grid-row-start: row가 어디에서부터 시작할지 지정
- 자식 요소에 지정
- row 얘기 X, Line 얘기
11. grid-row-end: row가 어디에서 끝날지 지정
- 자식 요소에 지정
- row 얘기 X, Line 얘기
12. grid-column: grid-column-start + grid-column-end
grid-column: [start line] / [end line];
- 맨 마지막 순서는 -1으로 표시, 마지막부터 -1, -2, -3...
- 위를 대신해서 span 사용 가능 ( [시작지점(생략 가능)] / span [차치하는 공간 수] )
- 지어준 line name들로도 사용 가능 (grid-column: [line name1] / [line name 2])
13. grid-row: grid-row-start + grid-row-end
grid-row: [start line] / [end line];
- 맨 마지막 순서는 -1으로 표시, 마지막부터 -1, -2, -3...
- 위를 대신해서 span 사용 가능 ( [시작지점(생략 가능)] / span [차치하는 공간 수] )
14. grid-template: grid-template-rows + grid-template-columns + grid-template-areas
- grid-area 적용한 뒤 사용해야 함
- grid-template-areas와 비슷하게 사용하지만 row의 높이, column의 너비를 지정해줘야 함
- 각 요소들이 나열된 부분의 앞뒤에 이름을 붙여줄 수 있음 ([start] "header header header header" 1fr [end])
- repeat는 사용 불가능
grid-template:
"header header header header" 1fr [row의 높이]
"content content content nav" 2fr [row의 높이]
"footer footer footer footer" 1fr [row의 높이] / 1fr 1fr 1fr 1fr [column들의 너비];
15. justify-items: 수평 (column 정렬)
- 개별 요소들, 각 cell들마다 적용
- 기본값 stretch (grid는 자식요소들을 늘여서 cell을 채우는 구조)
- 값을 바꾸면 자식요소들은 더이상 cell 전체를 커버하지 않음
- stretch가 아닐때 너비는 자식요소의 content 너비
- contnent가 없다면 화면에 아무것도 표시 X, 자식요소에 width & height 적용되어 있다면 표시됨
16. align-items: 수직 (row 정렬)
- 개별 요소들, 각 cell들마다 적용
- 기본값 stretch (grid는 자식요소들을 늘여서 cell을 채우는 구조)
- 값을 바꾸면 자식요소들은 더이상 cell 전체를 커버하지 않음
- stretch가 아닐때 높이는 자식요소의 content 높이
- contnent가 없다면 화면에 아무것도 표시 X, 자식요소에 width & height 적용되어 있다면 표시됨
17. place-items: justify-items + align-items
place-items: [align-items(수직) 값] [justify-items(수평) 값];
18. justify-content: 수평 (column 정렬)
- grid 전체에 적용, grid 자체를 움직인다고 생각
- stretch는 width, height이 fr이나 %로 적용되었을 때만 적용
19. align-content: 수직 (row 정렬)
- grid 전체에 적용, grid 자체를 움직인다고 생각
- stretch는 width, height이 fr이나 %로 적용되었을 때만 적용
19. place-content: justify-content + align-content
place-content: [align-content(수직) 값] [justify-content(수평) 값];
20. justify-self: justify-items의 개별 요소 적용 버전
- 자식요소에 적용
21. align-self: align-items의 개별 요소 적용 버전
- 자식요소에 적용
22. place-self: justify-self + align-self
- 자식요소에 적용
place-self: [align-self(수직) 값] [justify-self(수평) 값];
23. grid-auto-rows
grid-auto-rows: [row의 높이];
- 서버에서 가져온 내용의 숫자를 알 수 없을 때 사용
- grid-template-rows로 정해준 row의 숫자보다 더 많은 content가 있을 때 자동으로 row들을 더 만들어주는 역할
24. grid-auto-flow
- grid가 끝났지만 content가 더 있을 때 css는 그 content들을 담기 위해 사이즈 없는 여분의 div 넣음 (default)
- 위의 방식처럼 새 row를 넣기보다는 새 column을 넣을 수도 있음 (이 경우에는 content의 순서가 바뀜, flexbox처럼)
25. grid-auto-columns
grid-auto-columns: [columns의 너비];
- 서버에서 가져온 내용의 숫자를 알 수 없을 때 사용
- grid-auto-flow: column 과 함께 사용
- grid-template-columns로 정해준 column의 숫자보다 더 많은 content가 있을 때 자동으로 column들을 더 만들어주는 역할
#### keywords
1. minmax
- element가 얼마나 커질 수 있는지, 얼마나 작아질 수 있는지 저장
- element가 가능한 한 크기를 바라지만 너무 작아지지 않기를 원할 때 사용
- minmax(min, max)
- grid-template-columns: repeat(5, minmax(100px, 1fr)); 형태로 사용
: 100px보다 작아질 수 없고 1fr보다 커질 수 없음
2. auto-fit
- repeat function에만 사용
- element들을 늘려서 현재의 화면에 딱 맞도록 해 줌
- 5개의 element가 있을 때 이것들을 늘려서 화면 끝까지 5개의 element로 채움
- 반응형 디자인에서 중요!
- 혹은 items의 수를 미리 알 수 없을 경우
3. auto-fill
- repeat function에만 사용
- 특정 숫자 적어주는 대신에 사용
- 주어진 사이즈 내에서 column 만듦
- 5개의 element가 있을 때 화면 끝까지 다 차지 않았을 때 화면의 끝까지 빈 cell들을 만듦
- 가능한 한 많은 column들로 row를 채움
4. min-content
- 박스를 content가 필요한 만큼만 작게 만듦
- content가 얼마나 작게 만들어질 수 있는가
- repeat, minmax와 함께 사용가능 (반응형 디자인!)
5. max-content
- 박스를 content가 필요한 만큼 크게 만듦
- content가 얼마나 크게 만들어질 수 있는가
- repeat, minmax와 함께 사용가능 (반응형 디자인!)
'CSS' 카테고리의 다른 글
SCSS concepts (0) | 2020.04.21 |
---|